The Power of Functional Programming
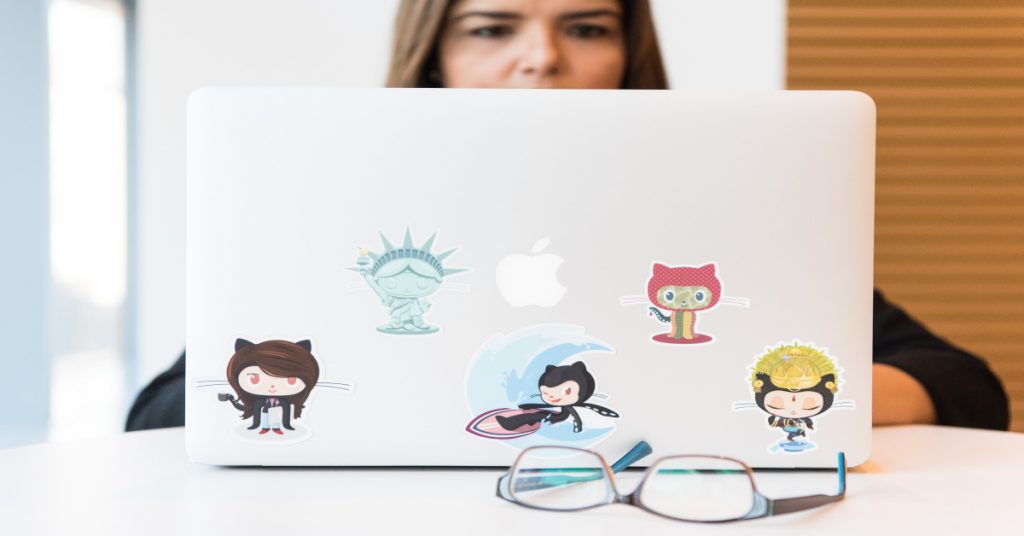
The Functional programming paradigm treats computation as the evaluation of mathematical functions and avoids changing state and mutable data.
The window.requestIdleCallback Function in JavaScript
Calling this function we should pass over the function we want to be invoked in the background in those moments when the one and only thread is free (not busy with other tasks). <!DOCTYPE html> <html lang=”en”> <head> <meta charset=”UTF-8″> <title>Title</title> </head> <body> <script> function doInBackground() { console.log(“doing work in background”); } if (window.requestIdleCallback) { […]
Binding Objects with Functions in ECMAScript 2016
The development ECMAScript 2016 has already started. One of the coming new capabilities will allow us to bind a function to specific object in order to be notified of changes that take place in that object. This capability is already supported in Google Chrome! This following code sample shows this capability in action. You can […]
Function as First Class Objects PRO
Similarly to JavaScript, we can pass over functions as objects. We can pass a function as a argument to a another function and we can store a function in a variable. The possibility to define anonymous functions and pass them over as arguments to other functions simplifies the code. goodMorning(str) => print(“Good Morning $str”); goodEvening(str) […]
The usort Function in PHP PRO
The usort function allows us to specify the criteria by which the array will be sorted. The following code sample includes the definition of the class Student and the creation of an array that its values are references for objects instantiated from Student. Using the usort function we can sort the students according to their […]
MooTools Function Binding PRO
Functions and Anonymous Classes in Scala PRO
When calling a function we indirectly invoke the apply method on the object that represents the function. We can use the anonymous inner class syntax for defining a new anonymous inner class that extends the Function relevant trait in order to create a new function. The following code sample shows that. object HelloSample { def […]
Functions as Objects in Scala PRO
Function values are treated as objects. The function A=>B is an abbreviation for using a new object instantiated from a class that extends the scala.Function1[A,B] trait and overrides the apply function. There are currently Function1, Function2, Function3… etc… up to Function22, that takes 22 parameters. The following code sample shows that. object HelloSample { def main(args:Array[String]):Unit […]
Generic Functions in Scala PRO
The Scala programming language allows us to define generic functions. This code sample shows that. object Sample { def main(args:Array[String]):Unit = { val ob = getMyStack[Int](10) } def getMyStack[T](num:Int) = { new MyStack[T](num) } } class MyStack[T](size:Int) { //… } The following video clips overviews this code sample, shows its execution and provides more information.
Function Type in Scala PRO
Scala allows us to define variables, function parameters and function returned values with a type that is a function. We can even specify the exact signature of that function. import annotation.tailrec object Program { def main(args: Array[String]):Unit = { var func:(Int,Int)=>Int = sum; println(func(4,3)) func = multiply println(func(4,3)) } def sum(a:Int,b:Int):Int = a+b def multiply(a:Int,b:Int):Int […]